Hibernate Annotation:
Hibernate configuration file
Hibernate is designed to operate in many different environments and, as such, there is a broad range of configuration parameters. SessionFactory is a global factory responsible for a particular database. If you have several databases, for easier startup you should use several <session-factory> configurations in several configuration files.
For full list of hibernate configuration properties Refer Chapter 1.1.4
<?xml version='1.0' encoding='UTF-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory name="factory"> <property name="connection.driver_class"> com.mysql.jdbc.Driver </property> <property name="connection.url">jdbc:mysql://localhost:3306/test</property> <property name="connection.username"> root </property> <property name="connection.password"> root </property> <property name="connection.pool_size">5</property> <!-- SQL dialect --> <property name="dialect"> org.hibernate.dialect.MySQLDialect </property> <!-- Echo all executed SQL to stdout --> <property name="hbm2ddl.auto">update</property> <mapping class="com.candidjava.hibernate.sample.dao.User" /> </session-factory> </hibernate-configuration>
Bean class
User.java
package com.candidjava.hibernate.sample.dao; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "std") public class User { @Id @Column(name = "id") @GeneratedValue(strategy = GenerationType.AUTO) private long id; @Column(name = "username") private String username; @Column(name = "password") private String password; public long getId() { return id; } public void setId(long id) { this.id = id; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
Dao class
What is SessionFactory?
You have to startup Hibernate by building a global org.hibernate.SessionFactory object and storing it somewhere for easy access in application code. A org.hibernate.SessionFactory is used to obtain org.hibernate.Session instances. A org.hibernate.Session represents a single-threaded unit of work. The org.hibernate.SessionFactory is a thread-safe global object that is instantiated once.
1. Usually an application has a single SessionFactory
2. SessionFactorys are immutable
3. The behaviour of a SessionFactory is controlled by properties supplied at configuration time
What is session?
The main runtime interface between a Java application and Hibernate. This is the central API class abstracting the notion of a persistence service.
The lifecycle of a Session is bounded by the beginning and end of a logical transaction. (Long transactions might span several database transactions.)
The main function of the Session is to offer create, read and delete operations for instances of mapped entity classes.
What is Transaction?
Allows the application to define units of work
UserDao.java
package com.candidjava.hibernate.sample.dao; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class UserDao { public static void main(String[] args) { User u = new User(); u.setUsername("Candidjava"); u.setPassword("123456"); SessionFactory factory = new Configuration().configure( "hibernate.cfg.xml").buildSessionFactory(); Session session = factory.openSession(); Transaction tx = session.beginTransaction(); session.save(u); tx.commit(); session.close(); System.out.println("record inserted"); } }
How to run:
Simply run the UserDao as Java application
Note: before running make sure hibernate Configuration is modified as per your system.
Library required:
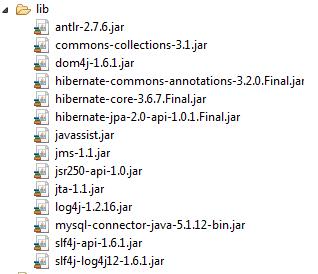
Download:
Download source code: